Top SQL Interview Questions For Freshers & Experienced 2025
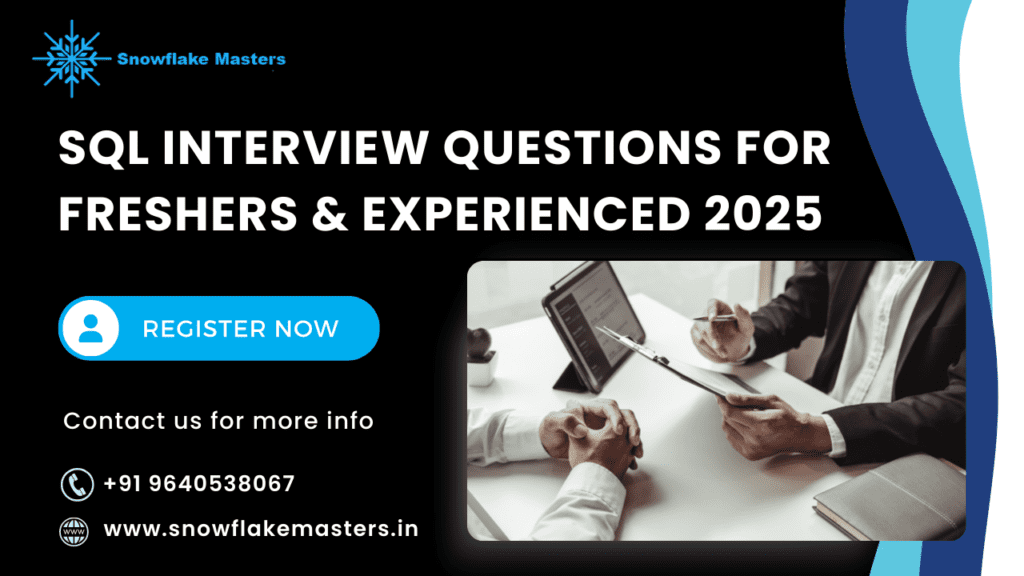
Sql Interview Questions For Freshers
1. What is SQL?
Answer: SQL (Structured Query Language) is the standard language used for managing and querying relational databases. As a fresher, understanding SQL fundamentals is key to answering SQL interview questions for freshers.
2. What are the types of SQL statements?
Answer: SQL statements are categorized as follows:
- DDL (Data Definition Language): CREATE, ALTER, DROP
- DML (Data Manipulation Language): SELECT, INSERT, UPDATE, DELETE
- DCL (Data Control Language): GRANT, REVOKE
- TCL (Transaction Control Language): COMMIT, ROLLBACK
These categories are often covered in SQL interview questions for freshers.
3. What is the difference between SQL and MySQL?
Answer: SQL is a language used for querying databases, while MySQL is an open-source relational database management system (RDBMS) that uses SQL for data management. This distinction is common in SQL interview questions for freshers.
4. What is a Primary Key?
Answer: A Primary Key is a column or set of columns in a table that uniquely identifies each row. It’s essential in SQL interview questions for freshers as it ensures data uniqueness.
5. What is a Foreign Key in SQL?
Answer: A Foreign Key is a column that links one table to another by referencing the Primary Key of another table, ensuring data integrity. Understanding Foreign Keys is important for SQL interview questions for freshers.
6. What is a JOIN in SQL?
Answer: A JOIN combines rows from two or more tables based on a related column. INNER JOIN, LEFT JOIN, and RIGHT JOIN are commonly tested in SQL interview questions for freshers.
7. What is the difference between INNER JOIN and LEFT JOIN?
Answer: A JOIN combines rows from two or more tables based on a related column. INNER JOIN, LEFT JOIN, and RIGHT JOIN are commonly tested in SQL interview questions for freshers.
8. What is the SELECT statement in SQL?
Answer: The SELECT statement retrieves data from one or more tables. It’s the most commonly used SQL operation, and understanding it is key for answering SQL interview questions for freshers.
9. What is the WHERE clause in SQL?
Answer: The WHERE clause is used to filter records based on a condition. It helps narrow down the result set, which is critical for SQL interview questions for freshers.
10. What is a Subquery in SQL?
Answer: A Subquery is a query embedded within another query. It can be used to filter or derive data for the outer query. Subqueries often appear in SQL interview questions for freshers.
11. What is the difference between UNION and UNION ALL?
Answer: UNION combines results from two queries, removing duplicates, while UNION ALL includes duplicates.
12. What are Aggregate Functions in SQL?
Answer: Aggregate functions operate on a set of values and return a single result. Examples include COUNT(), SUM(), AVG(), MIN(), and MAX(), which are key to SQL interview questions for freshers.
13. What is the GROUP BY clause?
Answer: The GROUP BY clause groups rows with the same values into summary rows.
14. What is the HAVING clause in SQL?
Answer: The HAVING clause is used to filter results after grouping with GROUP BY. It’s a common topic in SQL interview questions for freshers.
15. What is the difference between DELETE and TRUNCATE in SQL?
Answer: DELETE removes records based on a condition and can be rolled back, while TRUNCATE removes all rows from a table and cannot be undone.
16. What is an Index in SQL?
Answer: An Index is an object that speeds up data retrieval by allowing faster searches on a table’s columns. Indexing is often covered in Snowflake Training in Hyderabad Course
17. What is a View in SQL?
Answer: A View is a virtual table created by querying one or more tables. It does not store data but provides a simplified query result.
18. What is a Stored Procedure in SQL?
Answer: A Stored Procedure is a precompiled set of SQL statements that can be executed repeatedly. It helps improve performance and is a key topic for SQL interview questions for freshers.
19. What is a Trigger in SQL?
Answer: A Trigger is an automated action that runs in response to events like INSERT, UPDATE, or DELETE.
20. What is the difference between CHAR and VARCHAR in SQL?
Answer: CHAR is a fixed-length data type, while VARCHAR is a variable-length data type. This is a common distinction in SQL interview questions for freshers.
21. What is a Constraint in SQL?
Answer: A Constraint is a rule applied to data in a column to enforce data integrity, such as PRIMARY KEY, FOREIGN KEY, NOT NULL, and CHECK constraints. It’s a frequently asked topic in SQL interview questions for freshers.
22. What is the DISTINCT keyword in SQL?
Answer: The DISTINCT keyword removes duplicate rows from the result set, ensuring only unique records are returned.
23. What is the difference between ROLLBACK and COMMIT in SQL?
Answer: ROLLBACK undoes changes made during a transaction, while COMMIT saves changes permanently.
24. What is Normalization in SQL?
Answer: Normalization is the process of organizing data to reduce redundancy and improve data integrity.
25. What is Denormalization in SQL?
Answer: Denormalization involves combining tables to improve query performance, typically used in OLAP systems. Understanding this is important for SQL interview questions for freshers.
26. What is a Data Type in SQL?
Answer: A Data Type defines the type of data that can be stored in a column, such as INT, VARCHAR, DATE, and FLOAT.
27. What is a NULL value in SQL?
Answer: A NULL value represents missing or unknown data, and it’s crucial to differentiate from an empty string or zero.
28. What is the LIKE operator in SQL?
Answer: The LIKE operator is used for pattern matching in string values, often with wildcards like % and _.
29. What is a Self Join in SQL?
Answer: A Self Join is when a table is joined with itself. It’s commonly used with an alias for differentiation and is a key topic in SQL interview questions for freshers.
30. What is the difference between IN and EXISTS in SQL?
Answer: IN checks if a value is in a list, while EXISTS checks if a subquery returns any rows.
31. What is a CASE statement in SQL?
Answer: The CASE statement is used for conditional logic, similar to an IF-ELSE statement, and is commonly asked in SQL interview questions for freshers.
32. What is a Composite Key in SQL?
Answer: A Composite Key is a combination of two or more columns that uniquely identifies each row in a table.
33. What is a Normal Form in SQL?
Answer: A Normal Form is a set of guidelines used to organize a database efficiently, with the first, second, and third normal forms being most common.
34. What is the COUNT() function in SQL?
Answer: The COUNT() function returns the number of rows in a result set, commonly used in queries to count records based on conditions.
35. What is the difference between a View and a Table?
Answer: A Table stores actual data, while a View is a virtual table generated by a query.
36. What is a Temporary Table in SQL?
Answer: A Temporary Table exists only during a session
or transaction and is automatically dropped once the session ends.
37. What is an ALIAS in SQL?
Answer: An ALIAS is a temporary name given to a table or column for the duration of a query, simplifying complex queries.
38. What is the purpose of the ORDER BY clause in SQL?
Answer: The ORDER BY clause sorts the result set based on specified columns, in either ascending or descending order. This is a common question in SQL interview questions for freshers.
39. What is the difference between UNION and INTERSECT in SQL?
Answer: UNION combines results from two queries, while INTERSECT returns only the rows common to both queries. This distinction is key in SQL interview questions for freshers.
40. What is the EXCEPT operator in SQL?
Answer: The EXCEPT operator returns rows from the first query that are not present in the second query.
41. What is an Index and its types in SQL?
Answer: An Index is a data structure that enhances query performance. Types include Unique Index, Composite Index, and Clustered Index, common in SQL interview questions for freshers.
42. What is a database schema in SQL?
Answer: A Schema is a collection of database objects such as tables, views, and indexes, providing structure to the database.
43. What is the difference between a Trigger and a Stored Procedure?
Answer: A Trigger automatically executes in response to events, while a Stored Procedure is executed manually. This comparison is frequently asked in SQL interview questions for freshers.
44. What is a Data Warehouse in SQL?
Answer: A Data Warehouse is a system used for reporting and data analysis, storing large volumes of historical data.
45. What is a schema in SQL?
Answer: A Schema defines the logical structure of a database, including its tables, columns, and relationships.
46. What is an RDBMS in SQL?
Answer: An RDBMS (Relational Database Management System) stores data in relational tables. Examples include MySQL, Oracle, and SQL Server, which are common topics in SQL interview questions for freshers.
47. What is an aggregate function in SQL?
Answer: An Aggregate Function performs a calculation on a set of values and returns a single value, such as COUNT(), SUM(), and AVG().
48. What is a correlation name in SQL?
Answer: A Correlation Name is an alias for a table used in a query, particularly with joins and subqueries.
49. What are the different types of indexes in SQL?
Answer: Types of indexes include Unique Index, Clustered Index, and Non-clustered Index, all important for understanding performance optimization in SQL interview questions for freshers.
50. What are the advantages of using SQL?
Answer: SQL provides advantages like easy querying, robust data manipulation, and support for relational databases, making it a valuable skill in answering SQL interview questions for freshers.
SQL Interview Questions for Experienced Professionals
1. What is the difference between a Clustered and Non-Clustered Index?
Answer:
- Clustered Index: Stores and sorts table data based on the key values. A table can have only one clustered index.
- Non-Clustered Index: Maintains a separate structure and stores pointers to data rows, allowing multiple non-clustered indexes per table.
2. What is the purpose of a Unique Key in SQL?
Answer:
A Unique Key ensures all values in a column are distinct. Unlike a Primary Key, a table can have multiple Unique Keys, and they allow NULL values.
3. How does indexing impact database performance?
Answer:
Indexes speed up data retrieval but can slow down data modifications (INSERT, UPDATE, DELETE) due to the overhead of maintaining the index.
4. What is the use of the COALESCE() function in SQL?
Answer:
COALESCE() returns the first non-NULL value from a list of expressions. It’s often used to handle NULL values.
5. What are SQL Server CTEs, and why are they used?
Answer:
A Common Table Expression (CTE) is a temporary, named result set within a query. It simplifies complex queries and improves code readability.
6. What are the ACID properties in SQL?
Answer:
- Atomicity: Transactions are all-or-nothing.
- Consistency: Maintains database integrity.
- Isolation: Transactions don’t interfere with each other.
- Durability: Changes are permanent after commit.
7. What is the difference between DELETE, TRUNCATE, and DROP?
Answer:
- DELETE: Removes rows conditionally; can be rolled back.
- TRUNCATE: Removes all rows quickly; cannot be rolled back.
- DROP: Deletes the entire table structure.
8. How do you optimize SQL queries for performance?
Answer:
- Avoid using SELECT *.
- Use indexed columns for filtering.
- Optimize JOINs and avoid unnecessary subqueries.
- Analyze query execution plans.
9. What is the difference between SQL Server and PostgreSQL?
Answer:
SQL Server is a proprietary database from Microsoft, while PostgreSQL is open-source, known for its extensibility and advanced data type support.
10. What is a stored procedure, and how does it improve performance?
Answer:
A stored procedure is a precompiled collection of SQL queries. It reduces query parsing time and ensures reusability.
11. What are Window Functions in SQL?
Answer:
Window functions like ROW_NUMBER(), RANK(), and SUM() perform calculations across a set of table rows related to the current row.
12. What is the difference between WHERE and HAVING clauses?
Answer:
- WHERE: Filters rows before aggregation.
- HAVING: Filters aggregated data after a GROUP BY.
13. What is the difference between UNION and UNION ALL?
Answer:
- UNION: Combines result sets and removes duplicates.
- UNION ALL: Combines result sets without removing duplicates.
14. What are Temp Tables, and when do you use them?
Answer:
Temporary tables store data temporarily within a session. They are helpful for storing intermediate results in complex queries.
15. What is SQL Profiler, and how is it used?
Answer:
SQL Profiler is a tool to monitor SQL Server events, helping optimize queries and troubleshoot issues.
16. How do you handle deadlocks in SQL?
Answer:
- Optimize query design to reduce lock contention.
- Use appropriate transaction isolation levels.
- Identify and resolve deadlocks with monitoring tools.
17. What is the use of a Cross Join?
Answer:
A Cross Join creates a Cartesian product of two tables, combining each row of the first table with all rows of the second.
18. What are materialized views, and how do they differ from regular views?
Answer:
Materialized views store the results physically, improving performance for repeated queries. Regular views are virtual and don’t store data.
19. What is Database Normalization?
Answer:
Normalization organizes tables to reduce redundancy and dependency, typically up to 3NF or higher for efficient database design.
20. What are transaction isolation levels in SQL?
Answer:
- Read Uncommitted: Allows dirty reads.
- Read Committed: Prevents dirty reads.
- Repeatable Read: Prevents non-repeatable reads.
- Serializable: Ensures complete isolation.
21. What is the difference between RANK() and DENSE_RANK()?
Answer:
- RANK(): Assigns rank with gaps for ties.
- DENSE_RANK(): Assigns consecutive ranks without gaps.
22. What is the role of the NOLOCK hint in SQL?
Answer:
The NOLOCK hint reads data without acquiring locks, reducing contention but risking dirty reads.
23. What is a surrogate key?
Answer:
A surrogate key is an artificial key used to uniquely identify records, typically auto-incremented.
24. How is a trigger different from a stored procedure?
Answer:
Triggers execute automatically in response to events, whereas stored procedures are manually executed.
25. What are the different types of joins in SQL?
Answer:
- INNER JOIN: Matches records in both tables.
- LEFT JOIN: Includes all records from the left table and matching records from the right.
- RIGHT JOIN: Includes all records from the right table and matching records from the left.
- FULL OUTER JOIN: Includes all records when
- CROSS JOIN: Produces a Cartesian product. there’s a match in either table.
26. What is the difference between DELETE CASCADE and DELETE SET NULL?
Answer:
- DELETE CASCADE: Removes related records automatically.
- DELETE SET NULL: Sets foreign key fields to NULL when a referenced record is deleted.
27. What is a Pivot Table in SQL?
Answer:
A Pivot Table transforms row-based data into column format for better data analysis.
28. What is the purpose of indexing on multiple columns?
Answer:
Composite indexes optimize performance for queries filtering by multiple columns.
29. What is a recursive CTE?
Answer:
A recursive CTE references itself to handle hierarchical data like organizational charts.
30. What is partitioning in SQL?
Answer:
Partitioning divides large tables into smaller segments for performance optimization and easier management.
31. What is sharding in SQL?
Answer:
Sharding distributes a database across multiple servers, enhancing scalability and performance.
32. What are OLAP and OLTP systems?
Answer:
- OLTP: Focuses on transactional workloads.
- OLAP: Focuses on analytical workloads.
33. What is the MERGE statement in SQL?
Answer:
MERGE allows performing INSERT, UPDATE, or DELETE operations in a single statement based on a condition.
34. How do you optimize joins in SQL?
Answer:
- Use indexed columns.
- Minimize the number of joins.
- Avoid unnecessary data in SELECT.
35. What is a covering index?
Answer:
A covering index includes all columns in a query, avoiding the need to access the table directly.
36. What is the difference between EXISTS and IN in SQL?
Answer:
- EXISTS: Returns true if a subquery returns any rows and stops processing as soon as a match is found.
- IN: Compares values against a list or subquery result set and processes all values before returning results.
37. What are Indexed Views in SQL?
Answer:
Indexed Views are materialized views that physically store the query result, improving performance for complex, repeated queries.
38. How do you use the APPLY operator in SQL?
Answer:
The APPLY operator allows invoking a table-valued function for each row of a table. It is commonly used with CROSS APPLY and OUTER APPLY.
39. What is the difference between Scalar and Table-Valued Functions?
Answer:
- Scalar Functions: Return a single value.
- Table-Valued Functions: Return a table as output.
40. What are Sparse Columns in SQL Server?
Answer:
Sparse columns optimize storage for columns with a high percentage of NULL values by using less space.
41. How do you perform database migration in SQL?
Answer:
Database migration involves transferring data from one database to another using tools like Data Migration Assistant (DMA), SSIS, or custom scripts.
42. What is Query Execution Plan Caching?
Answer:
SQL Server caches query execution plans to reuse them for identical queries, improving performance by avoiding recompilation.
43. What is the significance of Fill Factor in Indexes?
Answer:
Fill Factor determines the percentage of space filled in a page when an index is created or rebuilt. It leaves free space for future growth and reduces fragmentation.
44. What is Parameter Sniffing in SQL?
Answer:
Parameter sniffing occurs when SQL Server uses the parameter value passed during the first execution of a stored procedure to generate and reuse an execution plan, which may not always be optimal for subsequent executions.
45. How do you prevent SQL Injection attacks?
Answer:
- Use parameterized queries or prepared statements.
- Avoid dynamic SQL.
- Validate and sanitize user inputs.
- Implement proper access controls.
46. What is Query Hints in SQL?
Answer:
Query hints are options added to a query to influence the query optimizer, such as forcing the use of a specific index or setting MAXDOP (maximum degree of parallelism).
47. What are the different types of cursors in SQL?
Answer:
- Static Cursor: Creates a snapshot of the result set.
- Dynamic Cursor: Reflects changes made to the data.
- Forward-Only Cursor: Can only move forward in the result set.
- Keyset-Driven Cursor: Tracks a set of keys in the result set.
48. How do you manage database backups and recovery?
Answer:
- Schedule regular full, differential, and transaction log backups.
- Use database recovery models (Full, Simple, Bulk-Logged) as needed.
- Test restoration regularly to ensure backup integrity.
49. What is Query Optimization in SQL?
Answer:
Query optimization involves techniques like using indexes, simplifying queries, reducing the number of joins, and analyzing execution plans to improve query performance.
50. What is the significance of database partitioning schemes?
Answer:
Partitioning schemes define how data is distributed across partitions. They enhance query performance and make large datasets more manageable.